Button
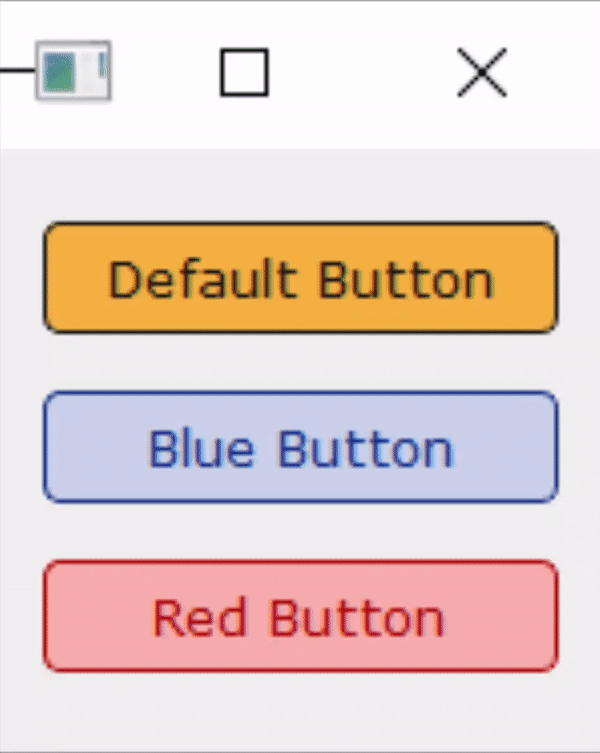
- class MangoUI.Button.Button(parent=None, primaryColor=(21, 21, 21, 255), secondaryColor=(245, 177, 66, 255), parentBackgroundColor=(240, 240, 240, 255), animationType=PyQt6.QtCore.QEasingCurve.Type.OutCubic, animationDuration=400, fontFamily='Verdana', fontSize=8, fontWeight='normal', borderStyle='solid', borderWidth=1, borderRadius=2)
Multi-colored, animated button.
- Parameters
parent (QObject, optional) – Parent object that contains this widget. Passing this ensures that destroying the parent also destroys this widget.
primaryColor (int, tuple, str or PyQt6.QtGui.QColor) –
Primary button color, as a 32-bit integer representing RGBA, tuple of floats representing RGBA values, HTML RGBA string, HTML hexadecimal string, or QColor object.
This is the default text color of the button and the background color of the button on hover.
secondaryColor (int, tuple, str or PyQt6.QtGui.QColor) –
Secondary button color, as a 32-bit integer representing RGBA, tuple of floats representing RGBA values, HTML RGBA string, HTML hexadecimal string, or QColor object.
This is the default background color of the button and the text color of the button on hover.
parentBackgroundColor (int, tuple, str or PyQt6.QtGui.QColor) –
Background color of parent widget, as a 32-bit integer representing RGBA, tuple of floats representing RGBA values, HTML RGBA string, HTML hexadecimal string, or QColor object.
The button border is set to this color on press and is used to provide an illusion of compression on press.
animationType (PyQt6.QtCore.QEasingCurve.Type) – Button animation type.
animationDuration (int) – Button animation duration in milliseconds.
fontFamily (str) – Font family of button text.
fontSize (int) – Font size of button text in point value.
fontWeight (str) – Font weight of button text.
borderStyle (str) – Border style of button.
borderWidth (int) – Border width of button in pixels.
borderRadius (int) – Border radius of button in pixels.
- animateBackground(currentBackgroundColor)
Update background color during animation.
- Parameters
currentTextColor (PyQt6.QtGui.QColor) – Current button background color.
- animateText(currentTextColor)
Update text color during animation.
- Parameters
currentTextColor (PyQt6.QtGui.QColor) – Current button text color.
- enterEvent(event)
Override enter event signal method for widget to set animation direction and start animation.
- Parameters
event (PyQt6.QtGui.QEnterEvent) – Event passed to base method.
- leaveEvent(event)
Override leave event signal method for widget to set animation direction and start animation.
- Parameters
event (PyQt6.QtCore.QEvent) – Event passed to base method.
- renderStyleSheet()
Set QSS style sheet for widget using defined attributes.
- setBorder(borderStyle=None, borderWidth=None, borderRadius=None)
Set button border properties.
- Parameters
borderStyle (str) – Border style of button.
borderWidth (int) – Border width of button in pixels.
borderRadius (int) – Border radius of button in pixels.
- setColors(primaryColor=None, secondaryColor=None, parentBackgroundColor=None)
Set button colors.
- Parameters
primary (int, tuple, str or PyQt6.QtGui.QColor) –
Primary button color, as a 32-bit integer representing RGBA, tuple of floats representing RGBA values, HTML RGBA string, HTML hexadecimal string, or QColor object.
This is the default text color of the button and the background color of the button on hover.
secondaryColor (int, tuple, str or PyQt6.QtGui.QColor) –
Secondary button color, as a 32-bit integer representing RGBA, tuple o floats representing RGBA values, HTML RGBA string, HTML hexadecimal string, or QColor object.
This is the default background color of the button and the text color of the button on hover.
parentBackgroundColor (int, tuple, str or PyQt6.QtGui.QColor) –
Background color of parent widget, as a 32-bit integer representing RGBA, tuple of floats representing RGBA values, HTML RGBA string, HTML hexadecimal string, or QColor object.
The button border is set to this color on press and is used to provide an illusion of compression on press.
- setFont(fontFamily=None, fontSize=None, fontWeight=None)
Set button text font properties.
- Parameters
fontFamily (str) – Font family of button text.
fontSize (int) – Font size of button text in point value.
fontWeight (str) – Font weight of button text.
- setupAnimationColors()
Set up button colors and animations.