Canvas
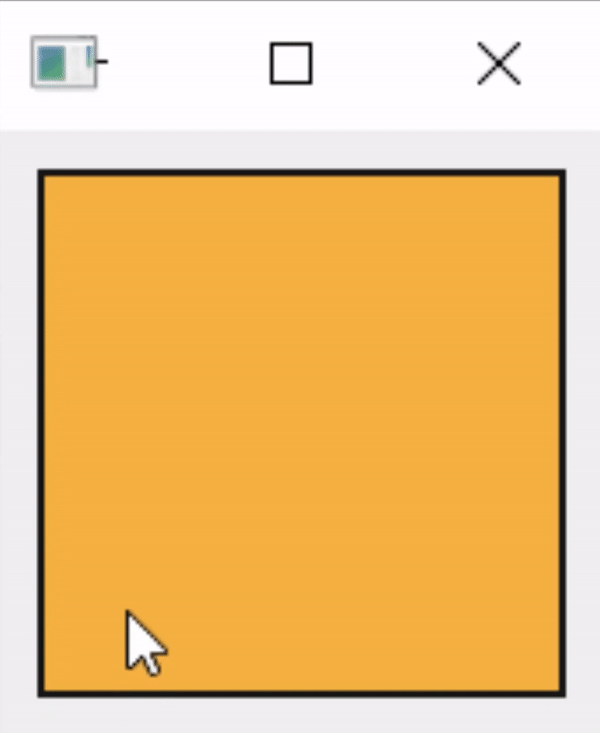
- class MangoUI.Canvas.Canvas(parent=None, width=200, height=200, canvasColor=(255, 247, 242, 255), penColor=(25, 25, 25, 255), strokeStyle=PyQt6.QtCore.Qt.PenStyle.SolidLine, strokeWidth=3, borderStyle='solid', borderColor=(0, 0, 0, 255), borderWidth=1)
Canvas widget for drawing.
- Parameters
parent (QObject, optional) – Parent object that contains this widget. Passing this ensures that destroying the parent also destroys this widget.
width (int) – Canvas height.
height (int) – Canvas height.
canvasColor (int, tuple, str or PyQt6.QtGui.QColor) – Canvas background color, as a 32-bit integer representing RGBA, tuple of floats representing RGBA values, HTML RGBA string, HTML hexadecimal string, or QColor object.
penColor (int, tuple, str or PyQt6.QtGui.QColor) – Pen color, as a 32-bit integer representing RGBA, tuple of floats representing RGBA values, HTML RGBA string, HTML hexadecimal string, or QColor object.
strokeStyle (PyQt6.QtCore.Qt.PenStyle) – Pen stroke style.
strokeWidth (int) – Pen stroke width.
borderStyle (str) – Border style of canvas.
borderColor (int, tuple, str or PyQt6.QtGui.QColor) – Border color of canvas. as a 32-bit integer representing RGBA, tuple of floats representing RGBA values, HTML RGBA string, HTML hexadecimal string, or QColor object.
borderWidth (int) – Border width of canvas in pixels.
- clearCanvas()
Clear canvas contents.
- mouseMoveEvent(event)
Override mouse move event signal to draw on canvas.
- Parameters
event (PyQt6.QtGui.QMouseEvent) – Event passed to base method.
- mouseReleaseEvent(event)
Override mouse release event signal to draw on canvas.
- Parameters
event (PyQt6.QtGui.QMouseEvent) – Event passed to base method.
- renderStyleSheet()
Set QSS style sheet for widget using defined attributes.
- resize(width, height)
Override resize method to set up new pixmap everytime the window is resized.
- Parameters
width (int) – Widget width.
height (int) – Widget height.
- saveCanvas(dest)
Save canvas content to image file.
- Parameters
dest (str or PyQt6.QtCore.QIODevice) – Image file destination path.
- setBorder(borderStyle=None, borderColor=None, borderWidth=None)
Set canvas border properties.
- Parameters
borderStyle (str) – Border style of canvas.
borderColor (int, tuple, str or PyQt6.QtGui.QColor) – Border color of canvas. as a 32-bit integer representing RGBA, tuple of floats representing RGBA values, HTML RGBA string, HTML hexadecimal string, or QColor object.
borderWidth (int) – Border width of canvas in pixels.
- setPen(penColor=None, strokeStyle=None, strokeWidth=None)
Set pen properties.
- Parameters
penColor (int, tuple, str or PyQt6.QtGui.QColor) – Pen color, as a 32-bit integer representing RGBA, tuple of floats representing RGBA values, HTML RGBA string, HTML hexadecimal string, or QColor object.
strokeStyle (PyQt6.QtCore.Qt.QPenStyle) – Pen stroke style.
strokeWidth (int) – Pen stroke width.
- setupPixmap()
Create and set new pixmap.